Type | Read/write | Author | Availability |
Read | Finbourne | Provided with LUSID |
The Tools.Mustache
provider enables you to write a Luminesce query that applies Mustache templating to a dataset.
Note: The LUSID user running the query must have sufficient access control permissions to use this provider. This should automatically be the case if you are the domain owner.
You can use this provider in conjunction with other providers to return data in Mustache templating, see example 4.
Basic usage
Input tables
Tools.Mustache
can take in different kinds of variables and outputs a table of data which can take various forms:
Input | Output | Information | Syntax |
| Table of data with one row and column | Simple scalar variables which can be used within Mustache templates. See example 1. | |
| Table of data with one row and column | Full tables of data which can be used within Mustache templates. Requires a specific syntax to be able to iterate over rows. Using this syntax, column names can be specified within Mustache templates to return values. See example 2. | |
| Table of data with one row and column | A table with two columns ( | |
| Table of data with one or more rows and columns | Returns all columns specified in a multi-line result, plus an additional column containing the populated Mustache template for each row. See example 4. Also returns a nested table if |
Examples
Example 1: Applying Mustache templating to some data
In this example, we use a simple scalar variable with Tools.Mustache
to return a table of data with one column and one row which contains our completed Mustache template.
The table of data returned looks like this:
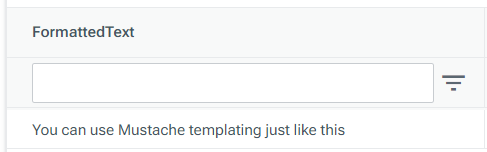
Example 2: Creating a Mustache template with a table of data
In this example, we create an input table of data which we use with Tools.Mustache
to create a Mustache template.
The table of data returned by the query looks like this, with the Mustache template populated with values:
Example 3: Creating a reusable partial Mustache template
You can use a special kind of variable called @partials
in your query to define a reusable partial Mustache template which you could use, for example, to create custom views that output a populated Mustache template.
In this example, we create a table of data and populate a @partials
variable with the required special syntax. We then use both variables with the Tools.Mustache
provider to return a single cell table of data.
The table of data returned by the query looks like this, with the partial template populated with data from our @some_data
variable, all within the larger Mustache template:
Example 4: Creating a Mustache template which outputs multiple rows
In this example, we use a special kind of variable called @foreach
with the Tools.Mustache
provider to return all columns and rows in the table, plus an additional column containing a populated Mustache template for each row.
The first ten rows of the table of data returned by the query looks like this:
Example 5: Creating a Mustache template which outputs a nested table
In this example, we first define a @foreach
variable which uses the group_concat
aggregate function with a data field. This variable is then used with Tools.Mustache
to create a Mustache template that outputs a nested table in an additional column.
Note the specific syntax used in @mustache_txt
. When constructing the Mustache message, AsTable
is appended to the column we want to format as a nested table. Within the column which is to become a nested table, fields are split on ,
and each field is referred to by its order number e.g., {{1}}
, {{2}}
.
The first ten rows of the table of data returned by the query looks like this: